Harness AI to dramatically speed up your content creation
Our AI-powered tool can generate high-quality, tailored content in seconds, not hours. Boost your productivity and focus on what really matters.
How to use the Python Code Generator
Python Code Templates & Examples
Check out these other templates
HTML&CSS Code Generator
Generate HTML and CSS code snippets with ease.
Java Code Generator
Generate Java code snippets with ease.
Chat with MARKy
Easily create content by just chatting with AI.
AI Text-to-Speech
Generate audio from text using AI. Supports 30+ languages.
AI Art & Images
Generate stunning images and art with AI. Express ideas. Stand out.
AI Photo Generator
Generate realistic photos with AI. Create custom images for any purpose.
AI Headshot Generator
Generate professional headshots with AI. For LinkedIn, email signatures, and more.
AI Interior Design Generator
Brainstorm interior design ideas at a fraction of the cost of hiring an interior designer.
Custom Generator
Generate custom text for any purpose.
Free AI Image Upscaler
Use our free tool to upscale your images and improve the quality of your photos.
AI Transcription
Transcribe and summarize audios. Generate titles, descriptions and show notes.
One Click Article Generation
Generate SEO-friendly articles with one click.
Create Faster With AI.
Try it Risk-Free.
Stop wasting time and start creating high-quality content immediately with power of generative AI.
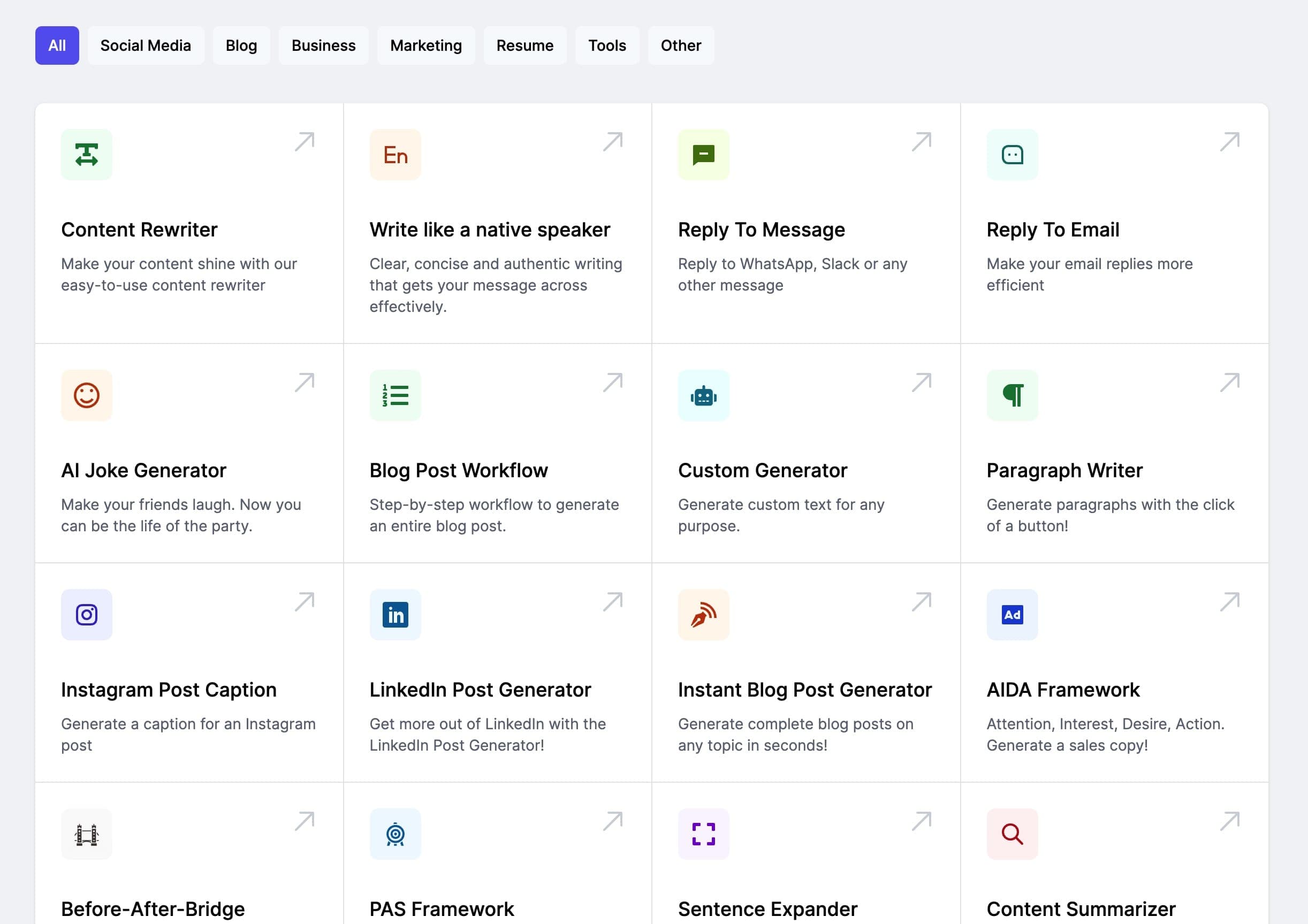